Venturing into the realm of blockchain and smart contracts, one language stands out due to its purpose-driven design and robustness – Solidity. At the heart of Ethereum and other blockchain platforms, Solidity allows developers to craft intricate and secure smart contracts. This article serves as your comprehensive guide to understanding Solidity data types, the building blocks of smart contracts. Grasping these data types is pivotal for anyone aspiring to navigate through and innovate within the blockchain landscape.
Solidity is statically typed, meaning that the data type of each variable must be specified at the time of declaration, ensuring better error checking and cleaner code. This meticulous approach to variable declaration bolsters security – a paramount concern in blockchain development. As we explore these data types, remember that each serves a unique purpose and understanding their nuances is key to optimizing smart contract performance and security.
Whether you’re a budding programmer aspiring to delve into decentralized applications (DApps) or an experienced developer aiming to refine your understanding of blockchain, this guide is tailored for you. We will dissect each data type, unraveling their intricacies in a simple yet comprehensive manner. Embrace this journey into Solidity, and let’s demystify these fundamental components that make smart contracts not just functional but also formidable.
Value Types
Value Types in Solidity are a fundamental concept, forming the building blocks of data handling and manipulation within smart contracts. These types store data directly in their own location in memory, unlike reference types which store the address, or the “reference”, to the location of the data. Here’s a bit more context and detail on each of the value types:
1. Booleans (bool)
- Booleans are the simplest value type, representing truth values – true or false.
- In Solidity, control structures like if, while, and for use booleans as their conditions, making them integral in decision-making processes within the contract.
2. Integers (int / uint)
- Solidity provides integers of various sizes. For unsigned integers, uint8 to uint256 (in increments of 8 bits), and for signed integers, int8 to int256.
- The absence of a number after int or uint defaults to int256 or uint256 respectively, which are 256 bits wide.
- Solidity does not allow implicit type conversion for non-literal values. This means developers need to handle data types with care to avoid errors.
- Special attention is needed for arithmetic operations to avoid overflow and underflow, which can lead to security vulnerabilities. For instance, if you subtract from a uint variable that holds a value of zero, it will underflow and wrap around to its maximum value.
- Solidity 0.8.x and newer versions have built-in overflow and underflow checking, but for older versions, it’s common to use libraries like OpenZeppelin’s SafeMath to prevent these issues.
3. Addresses (address)
- Addresses are a fundamental part of Ethereum and Solidity. Each account, whether a contract or an external (user) account, has an address.
- The address type comes in two flavors: address and address payable. The latter is needed to send Ether to the address using the transfer or send methods, reflecting the security-focused design of the language.
- Addresses have members and methods to query for balances, send Ether, and more. For example, address.balance provides the balance of the Ether stored in the address, and address.transfer(amount) is used to transfer Ether to another address.
- Smart contracts often use addresses to keep track of users or other contracts with which they interact.
Understanding and using these types properly is crucial for writing secure and efficient smart contracts. Mismanagement of these types, especially integers and addresses, can lead to serious vulnerabilities, including security breaches and loss of funds. As such, a deep understanding of these types and the operations associated with them is a significant step towards mastering Solidity and smart contract development.
Also Read: Cryptography Vs Blockchain: A Detailed Comparison
Reference Types
In Solidity, the programming language used for writing smart contracts on the Ethereum blockchain, understanding data types is crucial for effective memory management and data structuring. Solidity categorizes data types into two main categories: value types and reference types. While value types hold the actual data and are stored directly on the stack, reference types are more complex as they store references to the data, not the data itself. This distinction is important for optimizing gas costs and ensuring the efficient execution of smart contracts. Let’s delve deeper into the primary reference types in Solidity:
1. Arrays
- Arrays are a collection of elements that are of the same type. In Solidity, you can have fixed-size arrays, where the size is known at compile time, and dynamically-sized arrays, where the size can change during execution.
- They are particularly useful for storing lists of data, like a list of user addresses or token balances.
- Arrays can be stored in memory or on the blockchain’s storage, and understanding the difference is vital for gas optimization and contract design.
2. Structs
- Structs are custom-defined types that allow you to group together different value types (like integers, addresses, and others) into a single unit.
- They are useful for representing complex data structures, like a user with multiple attributes (e.g., an address, balance, and membership status).
- Structs can contain value types and reference types, and understanding how to use them efficiently can impact the contract’s gas consumption and performance.
3. Mappings
- Mappings are like hash tables and provide a key-value store.
- They are virtually initialized, meaning every possible key exists by default and is mapped to a value whose byte-representation is all zeros.
- Mappings are only stored in storage (permanent storage on the blockchain), and they cannot be iterated over. This means you can’t loop through all elements in a mapping, and you should maintain separate data structures if you need enumeration.
When working with these reference types in Solidity, it’s important to understand how data is stored and accessed, as it directly impacts the contract’s gas usage and efficiency. For instance, storing data in memory is cheaper but temporary, while storing data on the blockchain (storage) is permanent but more expensive. Also, understanding the difference between modifying data in place and creating a new copy is crucial for managing data consistently and avoiding unexpected bugs.
Proper handling of reference types in Solidity requires not only understanding these types but also mastering how the Ethereum Virtual Machine (EVM) handles memory and storage, how gas is consumed for various operations, and the security implications of data handling, especially when dealing with external calls and user inputs.
Data Location – Storage, Memory, and Calldata
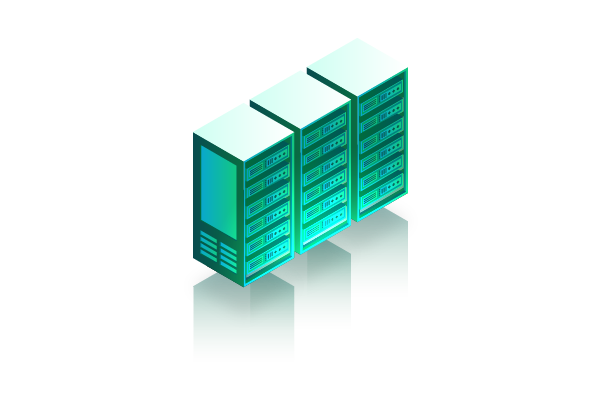
Solidity, the programming language primarily used for writing smart contracts on the Ethereum blockchain, introduces a concept known as “data location” to manage where and how data is stored during the execution of a smart contract. Understanding and optimizing data location is crucial for developers, not only to manage the state of the contract but also to optimize gas usage and enhance performance. The three primary data locations in Solidity are Storage, Memory, and Calldata:
1. Storage
- Purpose: Used to store state variables of the contract.
- Persistent: Data stored in Storage remains intact between function calls and even transactions, representing the contract’s state.
- Costly: Interacting with Storage is expensive in terms of gas costs. Reading from and writing to Storage are high-cost operations, reflecting the permanent nature of the data.
- Location: Stored on the Ethereum blockchain itself.
2. Memory
- Purpose: Provides a temporary place to store data during the execution of a function.
- Non-Persistent: Data in Memory is temporary and is erased between external function calls. It’s only available during the execution of a function.
- Cheaper: Using Memory is less expensive than using Storage because Memory data is not written to the blockchain. It’s an ideal place for temporary variables and intermediate calculations.
- Location: Stored temporarily during the execution of a function and not saved on the blockchain.
3. Calldata
- Purpose: Mainly used to hold function arguments.
- Read-Only: Unlike Memory, data in Calldata is immutable and cannot be modified during function execution.
- Gas-Efficient: It’s the most gas-efficient storage location when used for function inputs, especially with arrays and complex structures.
- External Visibility: Generally used for the parameters of external functions.
Balancing the use of Storage, Memory, and Calldata is a critical aspect of smart contract development in Solidity. Developers must make deliberate choices about data location to optimize for gas usage and performance. For instance, minimizing the number of state modifications (writes to Storage) and judicious use of Memory for temporary data can lead to more efficient smart contracts. Similarly, using Calldata for function inputs can reduce the gas cost of transactions, especially when passing arrays or complex data structures.
Understanding the nuances of these data locations allows developers to write optimized, cost-effective, and high-performance smart contracts on the Ethereum blockchain.
Also Read: What is Remix IDE? An Introduction to Ethereum Development
Special Data Types
Solidity, being a high-level language for Ethereum smart contracts, provides a set of specialized data types that cater to the unique needs of blockchain applications. In addition to basic types like integers and booleans, Solidity introduces more sophisticated data types to handle complex structures and operations efficiently. Here’s a deeper look into these special data types:
1. Bytes and Strings
- Bytes: This type is used to represent arbitrary-length fixed and dynamic byte data. bytes is a dynamic array of bytes, akin to byte[], but more cost-effective in terms of gas. Solidity provides bytes1 to bytes32 types, which consume less gas because they fit within the EVM’s word size of 32 bytes. Proper management of byte arrays is crucial, especially since operations like adding, removing, or slicing can be gas-intensive.
- Strings: Strings in Solidity are used for UTF-8 data, typically human-readable text. Internally, strings are stored and treated as variable-length byte arrays. However, they’re not as flexible or feature-rich as strings in languages like JavaScript or Python. For instance, string manipulation (concatenation, slicing, etc.) can be quite gas-expensive and lacks native string processing functions.
2. Enums (Enumerated Types)
Enums are a way to create user-defined types in Solidity. They are explicitly convertible to and from all integer types but implicit conversion is not allowed. An enum is used to define a variable that can only have one of few predefined values. These values are constants and are defined at compile time. Enums improve code readability, make it less error-prone, and express the intent more clearly. For example, you might use an enum to represent state values like Active, Paused, or InActive.
3. Function Types
Solidity treats functions as first-class citizens, meaning they can be assigned to variables, passed as arguments to other functions, and even returned from functions. This offers immense flexibility and allows for sophisticated constructs like higher-order functions. There are two types of function types in Solidity:
- Internal Function Types: These are functions that are only called internally from within the contract or from contracts deriving from it. They are not part of the contract’s interface. When an internal function is called, the code of that function is executed in the context of the calling contract.
- External Function Types: These functions are part of the contract’s interface, which can be called from other contracts and transactions. An external function call is essentially an EVM message call.
Understanding and utilizing these special data types effectively can lead to more optimized, readable, and secure Solidity smart contracts. However, it’s crucial to be mindful of the gas costs associated with operations involving these types, especially in a blockchain context where efficiency and optimization are paramount.
Error Handling
Error handling is a fundamental aspect of Solidity programming, as it helps in creating robust and secure smart contracts that operate reliably within the Ethereum ecosystem. Here’s a more detailed explanation of the error handling methods you’ve mentioned:
1. Assert
- Usage: It is primarily used to check for invariants and internal conditions that should never fail. If an assert statement fails, there’s likely a bug in the contract.
- Behavior: When an assert statement fails, it consumes all the remaining gas, and the transaction is reverted. This harsh penalty is deliberate to discourage developers from using assert for conditions that can be anticipated and handled more gracefully.
- Best Practices: It’s best used for conditions that should always be true and are not influenced by external factors, essentially serving as a sanity check.
2. Require
- Usage: It’s the go-to choice for input validation and conditional checks that depend on external inputs or contract state. If a condition is not met, it’s preferable to use require to validate it and revert the transaction if necessary.
- Behavior: When a require statement fails, it reverts the transaction and returns the remaining gas to the caller. This makes it a gas-efficient way to ensure conditions are met before executing further code.
- Best Practices: Use require for checking user inputs, contract state conditions, or the response from calling other contracts. It’s an effective way to ensure that functions behave as expected and to prevent contracts from reaching an invalid state.
3. Revert
- Usage: Similar to require, revert is used to halt the execution if a condition is not met. The key difference is that revert allows you to provide a custom error message, making it easier to understand why the transaction failed.
- Behavior: Just like required, it reverts the transaction and returns the unused gas. However, it provides an additional feature of custom error messages, which can greatly aid in debugging and providing more information to the users of the contract.
- Best Practices: Use revert when you want to provide detailed explanations about the reason for reverting the transaction. It’s especially useful in complex functions where you want to communicate specific reasons for the failure to the users or other contracts interacting with yours.
Mastering these error handling mechanisms is crucial for developing secure and efficient smart contracts. They not only prevent contracts from reaching an invalid state but also help in managing the contract’s gas consumption effectively. By using assert, require, and revert appropriately, developers can ensure their contracts behave predictably under all circumstances, making them more reliable and trustworthy in the decentralized environment of blockchain.
Conclusion
Understanding Solidity data types is more than a technical necessity; it’s a journey into the meticulous craft of smart contract development. From simple value types that form the backbone of contract logic to complex reference types that manage nuanced data structures, each data type is a thread in the intricate tapestry of blockchain applications. The wise management of data locations – storage, memory, and calldata – is not just a practice in optimization; it’s an art in balancing cost and efficiency.
In exploring the specialized data types and mastering error handling, developers don’t just write code; they sculpt resilient and efficient smart contracts capable of withstanding the demands of the decentralized world. This journey, laden with challenges and learning curves, is not merely about understanding a programming language. It’s about embracing a new paradigm of decentralized applications, where each line of code contributes to the grand vision of a transparent, secure, and inclusive digital future.
Solidity, with its structured approach to data types, offers a robust platform for this innovation. As you continue to explore and master Solidity data types, remember that each element, each type, and each structure you learn and apply, strengthens the foundation of this new world. Embrace the complexity, cherish the learning, and contribute to the ever-evolving landscape of blockchain technology.
Disclaimer: The information provided by HeLa Labs in this article is intended for general informational purposes and does not reflect the company’s opinion. It is not intended as investment advice or recommendations. Readers are strongly advised to conduct their own thorough research and consult with a qualified financial advisor before making any financial decisions.
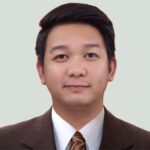
Joshua Soriano
I am Joshua Soriano, a passionate writer and devoted layer 1 and crypto enthusiast. Armed with a profound grasp of cryptocurrencies, blockchain technology, and layer 1 solutions, I've carved a niche for myself in the crypto community.
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link