Solidity, a cornerstone in blockchain development, is akin to JavaScript in its syntax yet boasts unique features tailored for smart contract creation. Smart contracts, the backbone of various decentralized applications, operate on blockchain platforms like Ethereum. These self-executing contracts encapsulate rules and automatically enforce them, ushering in a new era of trustless agreements.
Mapping in Solidity plays a pivotal role, akin to a dictionary or hash table in other programming languages. It offers an efficient, key-value store mechanism, crucial for managing data within smart contracts. Mapping’s ability to link unique keys to corresponding values underpins numerous blockchain functionalities, from tracking cryptocurrency balances to managing user permissions.
This article delves into the nitty-gritty of mapping in Solidity. We’ll explore its syntax, practical use cases, and best practices, providing you with a comprehensive understanding of this fundamental concept.
What is Mapping in Solidity?
Understanding mapping in Solidity is crucial for developing smart contracts on the Ethereum blockchain. In Solidity, a mapping is a data structure used to associate a key with a value, somewhat similar to dictionaries or hash maps in other programming languages. However, there are some unique characteristics and considerations when working with mappings in Solidity. Here are some key points to consider when it comes to understanding mappings in Solidity:
Key-Value Pairs
- Mappings store key-value pairs, where the key is unique within the mapping.
- In the example above, address is the key type, and uint256 is the value type. This mapping might be used to associate Ethereum addresses with their corresponding balances.
Initialization
- Mappings are not initialized by default, and you need to explicitly set values for specific keys.
- Accessing a key that has not been set will return the default value for the value type (e.g., 0 for uint256).
Also Read: What are Crypto Whitepaper and Litepaper? Key Elements and Differences
Storage vs. Memory Mappings
- Mappings can be declared as either storage or memory mappings, depending on where they are used.
- Storage mappings are written to the Ethereum blockchain and have persistent data that survives between transactions. They are typically used for state variables.
- Memory mappings exist only in the current function’s execution and are not stored on the blockchain. They are often used for temporary data storage within a function.
Restrictions
- Mappings cannot be iterated directly. You cannot loop through all the keys in a mapping.
- There is no way to determine the number of key-value pairs in a mapping, so you may need to manage this information separately if required.
Gas Costs
- Writing to a storage mapping consumes more gas than reading from it. Be mindful of gas costs when working with storage mappings, especially in loops or frequently called functions.
Key Deletion
- Solidity does not provide a built-in mechanism to delete a key from a mapping. To remove a key-value pair, you can set the value to its default (e.g., 0) or use a separate data structure to keep track of deleted keys.
Understanding these aspects of mappings in Solidity is crucial for designing efficient and secure smart contracts. Mappings are a fundamental tool for managing data in Ethereum contracts, and they play a significant role in various decentralized applications (DApps) and decentralized finance (DeFi) projects.
Syntax and Declaration
In Solidity, the syntax and declaration of mappings are essential concepts for managing data in smart contracts effectively. Mappings are a fundamental data structure that allows you to create relationships between keys and values in a way that is efficient and secure. Here’s a more detailed explanation of the syntax and declaration of mappings:
Keyword and Type Declaration
To declare a mapping in Solidity, you use the mapping keyword, followed by the key and value types enclosed in angle brackets (< >). The key type specifies what you’ll use as a lookup, and the value type defines the data associated with each key. For example, mapping(address => uint256) balances; declares a mapping named balances that associates Ethereum addresses (keys) with integer balances (values).
Supported Types
Solidity allows you to use a wide range of types as keys and values in your mappings. In the provided example, we used address as the key type and uint256 as the value type. However, you can use more complex types, such as:
- Strings: You can use strings as keys or values in mappings to associate string data with specific keys. For example, mapping(string => uint256) nameToScore; associates player names with their scores in a game.
- Structs: Mappings can also use user-defined structs as keys or values. This allows you to create more complex data structures. For instance, if you have a struct named Person with fields like name, age, and address, you can use mapping(uint256 => Person) personRecords; to associate unique IDs with individual Person structs.
Access and Modification
Once you’ve declared a mapping, you can access and modify its elements using the keys. For example, to access the balance of a specific Ethereum address in the balances mapping, you would use balances[address]. You can also update the balance by assigning a new value, like balances[address] = newBalance.
Initialization
Mappings in Solidity are not automatically initialized. You need to set values for individual keys explicitly. If you access a key that hasn’t been set before, it will return a default value (usually zero for numeric types). It’s essential to initialize mappings properly in your smart contract to avoid unexpected behavior.
Gas Costs
Mappings are efficient for lookups and updates because Solidity compiles them into a low-level data structure known as a hash table. However, be aware that modifying a mapping can consume gas, especially if you’re adding or updating many entries. Gas costs depend on the complexity of the operation and the data size.
Mappings in Solidity are a powerful tool for managing key-value relationships in smart contracts. They offer flexibility in choosing key and value types, making them suitable for a wide range of use cases, from tracking balances to more complex data structures like dictionaries and registries. Understanding the syntax and declaration of mappings is crucial for developing robust and efficient smart contracts on the Ethereum blockchain.
Practical Examples
In Solidity, mapping is a widely used data structure that allows you to associate a key with a value, similar to a dictionary or hash map in other programming languages. Here are some practical examples of how mappings can be used in Solidity:
Address to Balance Mapping
You can use a mapping to track the balances of Ethereum addresses within a smart contract. This is useful for creating wallets, financial applications, or any system where you need to manage account balances.
Voting System
Mappings can be employed to implement voting systems. Each candidate can be represented by a unique key, and the mapping stores the number of votes received by each candidate. This is useful for conducting fair and transparent voting processes.
Access Control
Mappings are crucial for access control within a smart contract. You can associate addresses with certain privileges, such as admin or user roles. This allows you to restrict access to specific functions or actions based on the user’s role.
Token Balances
Mappings are commonly used in token contracts to maintain the balances of token holders. Each Ethereum address is mapped to a token balance, enabling users to transfer tokens to each other securely.
Record Keeping
In various applications, mappings can be used for record-keeping purposes. For example, in a supply chain management system, you can use mappings to track the ownership or status of products at different stages.
Data Indexing
Mappings can serve as efficient data indexes. For instance, in a decentralized application (DApp) that manages user profiles, you can use a mapping to associate user IDs with their corresponding profile data.
Multi-Signature Wallets
Multi-signature wallets often use mappings to keep track of authorized signers and the number of required signatures to execute a transaction. Mappings help in managing the ownership and control of funds in such wallets.
User Permissions
In decentralized applications that involve user permissions and role-based access, mappings can be used to define roles and map users to their respective roles. This allows for fine-grained control over who can perform specific actions.
Game State
In decentralized games, mappings can be used to store game state information, such as player scores, game progress, and in-game assets owned by each player.
Registry Systems
Mappings can be employed in registry systems where you associate keys with specific information, such as domain names in a decentralized domain name system (DNS) or asset registration in a digital asset registry.
Mappings in Solidity are versatile and provide a flexible way to structure and access data within smart contracts, making them a fundamental tool for various decentralized applications and blockchain-based systems.
Advanced Mapping Techniques
Advanced mapping techniques in Solidity refer to the use of mappings, a fundamental data structure in the Ethereum blockchain’s programming language, Solidity, in more complex and nuanced ways to efficiently store and retrieve data on the blockchain.
Mappings are often used to create key-value pairs, where keys are unique identifiers, and values are associated data. Here are some advanced mapping techniques and concepts in Solidity:
Nested Mappings
You can create mappings within mappings, allowing you to build hierarchical data structures. For example, you could have a mapping that associates user addresses with their balances, and within each user’s balance mapping, you can store different types of assets as another mapping.
Mappings with Enumerations
Enumerations (enums) can be used as keys in mappings to create more structured data storage. For instance, you can use an enum to represent different states or categories and map them to associated data.
Mapping Iteration
Solidity does not natively support iterating over mappings due to the unpredictability of gas costs. However, you can implement your own iteration patterns by maintaining an array of keys or using libraries like IterableMapping (a community-contributed library) to iterate through mapping elements.
Mapping Storage vs. Mapping Memory
Solidity mappings can be declared as storage or memory variables, which affects how data is stored and accessed. Storage variables persist between function calls and modify the blockchain state, whereas memory variables are temporary and used for data manipulation within a function. Understanding when to use storage or memory is crucial for optimizing gas costs.
Mapping Deletion
Deleting data from a mapping is not straightforward. Solidity does not provide a built-in method to remove an element from a mapping. To delete a mapping entry, you often need to use a separate boolean flag to mark entries as deleted or rely on workarounds.
Gas Optimization
Careful consideration of gas costs is vital when working with mappings. Iterating over large mappings or using nested mappings can lead to high gas costs. Properly managing and minimizing gas usage is essential for efficient smart contract development.
These advanced mapping techniques in Solidity demonstrate how developers can design more complex data structures and optimize gas consumption in smart contracts. Solidity’s mapping data type is a powerful tool for managing data on the Ethereum blockchain, but it requires a deep understanding of its nuances to build efficient and secure decentralized applications (DApps).
Best Practices and Common Pitfalls
Mapping is a commonly used data structure in Solidity, the programming language for Ethereum smart contracts. It allows you to create key-value pairs, similar to dictionaries or associative arrays in other programming languages. While mappings are a powerful tool, they come with some best practices and common pitfalls you should be aware of:
Best Practices
- Use Mappings for Efficient Data Retrieval: Mappings are efficient for retrieving data based on a specific key. They have constant time complexity (O(1)) for both insertion and retrieval.
- Use Enumerations or Arrays for Iteration: Mappings are not iterable, so if you need to iterate through all the elements, consider using arrays or an enumeration pattern alongside mappings to keep track of keys.
- Initialize Mappings in Constructor: Initialize mappings in the constructor or an initialization function to ensure they start with the desired default values. Mappings are not automatically initialized to default values like arrays.
- Handle Key Non-Existence: Check whether a key exists in a mapping before accessing its value, as accessing a non-existing key in a mapping returns the default value for that data type (e.g., 0 for uint).
- Use a Struct for Complex Data: When dealing with complex data structures, consider using a struct as the value type in your mapping to keep your code organized and more readable.
- Access Control: Implement access control mechanisms to restrict who can modify the mapping. Use modifiers and access control functions to ensure only authorized users can update the mapping.
- Size Limitations: Be mindful of the gas costs associated with mapping storage. Excessive data in mappings can lead to high gas costs, which may make your contract less efficient.
Also Read: Public Key Vs. Private Key: A Comparative Guide
Common Pitfalls
- Reentrancy Vulnerabilities: Be cautious when using mappings in functions that interact with external contracts. Malicious external contracts can trigger reentrancy attacks. Use the “checks-effects-interactions” pattern to mitigate this risk.
- Large Mappings: Storing a large number of items in a mapping can increase gas costs and potentially make your contract unmanageable. Consider alternative storage solutions or pagination if you anticipate a large number of entries.
- Inefficient Key Generation: If you use dynamically generated keys, ensure that the key generation process is efficient. Inefficient key generation can lead to higher gas costs.
- Security Around Key Generation: Ensure that keys are generated securely to prevent unauthorized access to sensitive data. Avoid using predictable keys that an attacker could guess.
- Mapping Limitations: Mappings are not suitable for every use case. In some scenarios, other data structures like arrays or sets may be more appropriate.
- Lack of Error Handling: Always handle errors that might occur when interacting with mappings. Failing to check for errors can lead to unexpected behavior and vulnerabilities.
- Upgradeability: If you plan to upgrade your smart contract, consider the implications of modifying or changing the structure of existing mappings, as it can impact the contract’s compatibility with the old data.
Mappings are a fundamental part of Solidity, and when used correctly, they can be a powerful tool for managing data within your smart contracts. However, it’s essential to be aware of their limitations and potential pitfalls to write secure and efficient smart contracts.
Conclusion
In conclusion, mappings in Solidity are like a reliable Swiss Army knife for blockchain developers, offering a straightforward way to link keys with values. They’re the go-to tool for managing data in Ethereum smart contracts, enabling us to keep track of everything from cryptocurrency balances to voting records. But remember, while mappings are powerful, they come with their own quirks. You’ve got to initialize them, be mindful of gas costs, and handle potential pitfalls like reentrancy vulnerabilities or large data storage. So, when crafting your smart contracts, think of mappings as your trusty sidekick, always ready to help, but handle with care to ensure the security and efficiency of your blockchain creations.
Mappings are the building blocks that make decentralized applications and blockchain-based systems tick, giving us the ability to associate keys and values efficiently. They’re like the keys to various doors in the world of Solidity, allowing us to open up new possibilities for data management. So, as you embark on your journey into blockchain development, keep these mapping essentials in your toolkit, and you’ll be well on your way to crafting robust and efficient smart contracts.
Disclaimer: The information provided by HeLa Labs in this article is intended for general informational purposes and does not reflect the company’s opinion. It is not intended as investment advice or recommendations. Readers are strongly advised to conduct their own thorough research and consult with a qualified financial advisor before making any financial decisions.
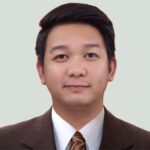
Joshua Soriano
I am Joshua Soriano, a passionate writer and devoted layer 1 and crypto enthusiast. Armed with a profound grasp of cryptocurrencies, blockchain technology, and layer 1 solutions, I've carved a niche for myself in the crypto community.
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link